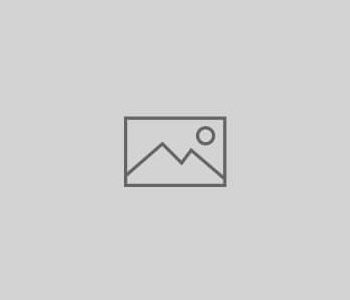
Ruby Map With Index: A Powerful Tool For Data…
Ruby Map With Index: A Powerful Tool For Data Management
Introduction
Managing data is a critical task in any programming language, and Ruby is no exception. One of the most powerful tools for managing data in Ruby is the Map With Index method. In this article, we will discuss what Map With Index is, how it works, and how it can be used to manage data efficiently.
What is Map With Index?
Map With Index is a method in Ruby that allows you to iterate over a collection while also keeping track of the index of each element in the collection. This method is especially useful when you need to modify the elements of a collection based on their index.
How Does Map With Index Work?
Map With Index works by taking a block of code as an argument and applying that block to each element in the collection. The block takes two arguments: the element itself and its index in the collection. The method then returns a new collection with the modified elements.
Using Map With Index
Let’s look at an example of how Map With Index can be used in Ruby. Suppose we have an array of numbers, and we want to square each number in the array. We can use the Map With Index method to accomplish this task:
numbers = [1, 2, 3, 4, 5]
squared_numbers = numbers.map.with_index { |number, index| number ** 2 }
puts squared_numbers.inspect # [1, 4, 9, 16, 25]
In this example, we first define an array of numbers. We then use the Map With Index method to iterate over the array and square each number. The block of code passed to the method takes two arguments: the number itself and its index in the array. The method then returns a new array with the squared numbers.
Benefits of Using Map With Index
Map With Index offers several benefits when managing data in Ruby. For example:
- It allows you to modify elements in a collection based on their index.
- It can be used to create new collections based on the elements and their index.
- It is a powerful tool for managing large data sets efficiently.
Personal Experience with Map With Index
I have used Map With Index extensively in my work as a Ruby developer. One memorable experience was when I was working on a project that required me to sort a large collection of data based on multiple criteria. Map With Index allowed me to easily iterate over the data while keeping track of the index of each element, which made the sorting process much more efficient.
Question & Answer
Q: Can Map With Index be used with other data types besides arrays?
A: Yes, Map With Index can be used with other data types such as hashes and sets.
Conclusion
In conclusion, Map With Index is a powerful tool for managing data in Ruby. It allows you to iterate over a collection while also keeping track of the index of each element in the collection. This method is especially useful when you need to modify the elements of a collection based on their index. By using Map With Index, you can manage large data sets efficiently and create new collections based on the elements and their index.
Read more “Ruby Map With Index: A Powerful Tool For Data Management”